Unlocking the Magic: Basic Python Components and Scripts for the CCNP 350-401 ENCOR Exam
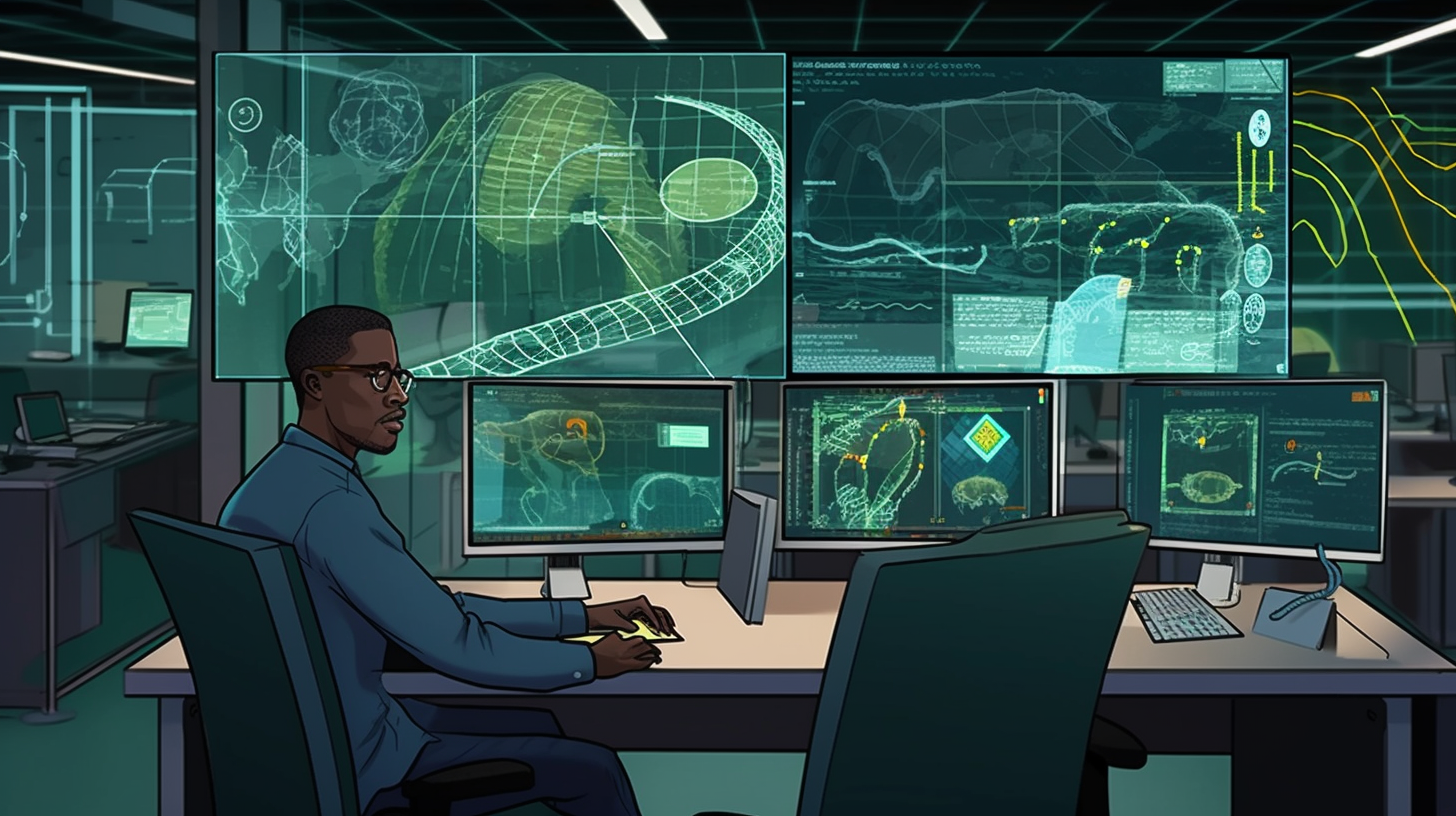
Welcome, dear reader! If you’ve found your way here, you’re likely on the arduous journey of preparing for the CCNP 350-401 ENCOR exam, right? Well, you’re in for a treat. Contrary to popular belief, the world of network engineering isn't just about untangling cables or furiously typing cryptic commands into a terminal. No, it’s also about mastering some fundamental Python concepts that are essential for dealing with modern-day network automation and programmability. Let’s dive into the Pythonic world and unearth the treasures within!
The Building Blocks: Basic Components of Python
Before we go conjuring up complex scripts, let’s start with the basics. Think of these components as the fundamental ingredients in a recipe for an exquisite digital feast.
1. Variables and Data Types
Ever wondered why some people call Python the “Swiss Army Knife” of programming languages? It’s mainly because of its simplicity and flexibility when handling data. Variables in Python are like little containers that hold data values. Whether you’re dealing with numbers, strings, or even that elusive unicorn emoji, Python’s got you covered.
Python’s primary data types include:
- Numbers: Integers, floating-point numbers, and complex numbers.
- Strings: A sequence of characters enclosed in quotes.
- Lists: Ordered and mutable collections of items.
- Tuples: Ordered but immutable collections of items.
- Dictionaries: Unordered and mutable collections of key-value pairs.
- Sets: Unordered collections of unique items.
Variables don’t need explicit declaration for type; they gracefully assume the type of the value assigned to them. It’s like the chameleon of coding.
2. Control Flow Statements
Ah, control flow statements—the traffic cops of your code. By dictating the flow of execution, these statements ensure your Python scripts don’t end up in a pile-up on the coding highway.
The key players are:
- If-Else: Makes decisions in your code. If one thing is true, do this; else, do that. Simple, right?
- For Loops: Iterate over sequences (like lists or strings) in a loop. Perfect for when you need to do something repeatedly.
- While Loops: Keep looping as long as a condition remains true. It's like being stuck in a roller coaster until the operator decides you’ve had enough.
Getting these right is like ensuring you have the perfectly synchronized gears in your watch. Miss one beat, and everything comes to a grinding halt.
3. Functions
Functions in Python are essentially the reusable chunks of code that can be called upon whenever needed. They can accept inputs, process them, and return outputs. Think of them as the efficient little worker bees of your code, tirelessly working to get your tasks done.
Here’s a simple example to tickle your curiosity:
def say_hello(name):
return f"Hello, {name}!"
print(say_hello('world'))
With just a few lines, you can make your code more modular and easier to manage. Magic, isn’t it?
Let’s Get Scriptural: Writing Basic Python Scripts
Now that we have the foundational pieces in place, it’s time to put them together in the form of scripts. Writing scripts can make your daily tasks as a network engineer so much easier, whether it’s automating configuration changes or even monitoring network performance. Let’s start with some basic examples and slowly crank up the complexity.
1. The Classic “Hello, World!” Script
Ah, the quintessential starting point of any programmer’s journey—the “Hello, World!” script. It’s like the first awkward hello at a party, an essential icebreaker.
print('Hello, World!')
There you have it! With just one line, you’ve embarked on your Python scripting adventure.
2. Automated Network Pinger
Okay, let’s step it up a notch. How about a script to ping multiple devices on your network? This little marvel will save you time and effort, making it an indispensable tool in your scripting arsenal.
import os
def ping_devices(device_list):
for device in device_list:
response = os.system(f"ping -c 1 {device}")
if response == 0:
print(f"{device} is up!")
else:
print(f"{device} is down!")
devices = ['192.168.1.1', '192.168.1.2', '192.168.1.3']
ping_devices(devices)
Easy, right? This script uses Python’s os.system
function to send ping commands and report on the status of each device. Pretty neat!
3. Fetching Data via APIs
If you’re in the network engineering domain, API calls are your new best friend. Many modern network devices provide RESTful APIs for interaction. Let’s script a basic example to fetch data from a web page using Python’s requests
library.
import requests
def get_page_content(url):
response = requests.get(url)
if response.status_code == 200:
return response.text
else:
return f"Failed to retrieve content. Status code: {response.status_code}"
url = 'http://example.com'
print(get_page_content(url))
With just a few lines, this script fetches the content of a web page and prints it. You can then parse this data to extract valuable information for your network operations.
Making It Amusing: When Python Scripts Don’t Go As Planned
Now, let’s take a breather and inject some humor into our scripting journey. If you’ve ever written code, you’ll know it’s not all rainbows and unicorns. Sometimes, things go hilariously wrong.
Picture this: You’ve written a Python script to automate backups of your network configurations. Everything seems fine—until it starts backing up every single file on your laptop, including that 2GB collection of cat memes. Now you’re sitting there, watching in horror as your system runs out of space while the world’s largest collection of funny cat videos gets uploaded to your network server.
Here’s a sneak peek into how you might accidentally set yourself up for a comedic disaster:
import shutil
import os
def backup_configurations(source_directory, backup_directory):
try:
shutil.copytree(source_directory, backup_directory)
print("Backup successful!")
except Exception as e:
print(f"An error occurred: {e}")
source = '/home/user/configs' # Oops! Too generic
backup = '/home/user/backup'
# Error: Forgot to check if paths are valid and correctly set up
backup_configurations(source, backup)
In the words of the great Pythonista, “with great power comes great responsibility.” Always double-check your scripts and, if possible, test them in a safe environment to avoid these unwelcome surprises. Your future self will thank you for it!
Scripting for Network Engineers: Real-World Use Cases
Alright, it’s time to get back on track and discuss some real-world applications of Python scripts in network engineering. These scripts can help you automate repetitive tasks, monitor network health, and even troubleshoot issues more efficiently. Let’s explore a few more sophisticated examples.
1. Automating Device Configuration
One of the most common tasks network engineers face is configuring multiple devices. Doing this manually is not just tedious but also prone to human error. Let’s script a way to push configurations to multiple devices using Python.
import paramiko
def configure_device(host, username, password, config_commands):
ssh = paramiko.SSHClient()
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
try:
ssh.connect(host, username=username, password=password)
for command in config_commands:
stdin, stdout, stderr = ssh.exec_command(command)
exit_status = stdout.channel.recv_exit_status()
if exit_status == 0:
print(f"Command succeeded: {command}")
else:
print(f"Failed to execute command: {command}")
ssh.close()
except Exception as e:
print(f"An error occurred: {e}")
devices = [
{'host': '192.168.1.1', 'username': 'admin', 'password': 'pass'},
{'host': '192.168.1.2', 'username': 'admin', 'password': 'pass'}
]
commands = [
'interface GigabitEthernet0/1',
'ip address 192.168.2.1 255.255.255.0',
'no shutdown'
]
for device in devices:
configure_device(device['host'], device['username'], device['password'], commands)
This script uses the paramiko
library to establish SSH connections to network devices and push a set of configuration commands. Automation at its finest!
2. Network Monitoring and Alerts
Keeping an eye on network performance is crucial for maintaining optimal operations. Python can help you monitor key performance indicators and even send alerts when thresholds are breached.
import psutil
import smtplib
from email.mime.text import MIMEText
def check_network_usage():
net_io = psutil.net_io_counters()
sent = net_io.bytes_sent / (1024 * 1024) # Convert to MB
recv = net_io.bytes_recv / (1024 * 1024) # Convert to MB
return sent, recv
def send_alert(message):
msg = MIMEText(message)
msg['Subject'] = 'Network Usage Alert'
msg['From'] = 'admin@example.com'
msg['To'] = 'admin@example.com'
try:
with smtplib.SMTP('smtp.example.com') as server:
server.login('admin@example.com', 'password')
server.send_message(msg)
print("Alert sent!")
except Exception as e:
print(f"Failed to send alert: {e}")
sent, recv = check_network_usage()
if sent > 1000 or recv > 1000: # Arbitrary threshold of 1GB
alert_message = f"High network usage detected! Sent: {sent} MB, Received: {recv} MB"
send_alert(alert_message)
Here, the script uses the psutil
library to gather network usage statistics and sends an email alert if the usage exceeds a specified threshold. This ensures you’re always in the loop.
The Py-Ending: Wrapping It All Up
There you have it—a whirlwind tour through the basic components and scripting capabilities of Python tailored for aspiring CCNP 350-401 ENCOR exam takers. From the essential building blocks like variables and control flow statements to practical scripts for network engineering tasks, we’ve covered a lot of ground. And, let’s not forget the occasional hilarity that ensues when scripts take an unexpected turn.
Remember, the journey of mastering Python is a marathon, not a sprint. Keep experimenting, breaking things, and learning along the way. Your reward? A seamless, automated, and efficient network operation synonymous with the magic of Python. So go forth and code, because in the end, it’s all just a bunch of ones and zeros!
Good luck on your exam and happy scripting!